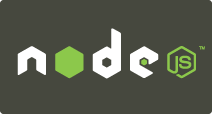
What is Node.js? It's a general-purpose programming platform using JavaScript as the language, and a runtime full of asynchronous file-system and network I/O functions. It supports an event-oriented model of programming, using JavaScript features like anonymous callback functions and Promise objects to their fullest. It is a lightweight system that eschews traditional thread-based architectures, and instead relies on a single threaded event-loop architecture. The combination produces a system that can sustain very high transaction throughput, and is very easy to program for.
Spring is kind of the antithesis to Node.js, and it may be that Node.js's popularity is due to many programmers tired of the over-the-top nature of the Spring ecosystem. What I mean is that Spring throws in dozens of kitchen sinks worth of features to make an extremely complicated system that tries to be the be-all-end-all. To be fair, many of the kitchen sinks I refer to are not Spring per-se but other projects like Hibernate and Java Persistence. Through Spring DAO objects, a specific MVC (Model View Controller) model is strongly encouraged. The system is written in Java, on Java EE appservers like Tomcat or Glassfish. These appservers are themselves large hairy beasts full of complexity, and strongly dependent on a thread-based architecture.
Maybe that characterization of Spring will offend some. Consider that 10+ years ago I worked at Sun in the Java SE team, and was routinely writing blog posts on my java.net blog defending Java as being superior to JavaScript. Today my opinions have flipped because of my experience with Node.js, and the ease of programming it offers. I currently am employed writing Spring code ... the other day I needed to return a JSON object to an HTTP POST request, and therefore needed to create a JSON Mapper object -- because -- uh -- Spring makes you do all kinds of excess work to get anything done. Namely, I needed to create a Java class with a couple dozen fields. After using Eclipse to auto-generate the Constructor, the Getters and Setters, and the hashCode and toString and equals methods, the class weighs in at almost 600 lines of code. All that just to manage a couple dozen fields.
By contrast in Node.js the same task - returning JSON from an HTTP POST request - would take a handful of lines of code. Rather than be burdened with implementing a 600 line monstrosity just to return some data, in Node.js you simply pass an anonymous object (because JavaScript supports such things) to a method that formats objects as JSON, and QED the thing is done as quick as can be.
This was a slight detour from answering the question, but it points in the direction I want to take. Namely - Node.js is full of low computational cost solutions that are easy to code, easy to maintain, and straightforward. Spring, on the other hand, is full of heavy-weight solutions because what seems to me as a mindset that Spring (and the whole Java ecosystem) goes beyond the pale to solve every last problem that exists.
Node.js does not have any opinion about how you organize your code. It doesn't care where the source files are located, what's in them, whether you define proper classes, whether you use anonymous objects, whether the capitalization of method or variable or class names is correct, whether you use the model-view-controller paradigm, and on and on and on. Its role is to simply provide a base set of runtime modules, and an excellent JavaScript engine sourced from the Google Chrome team. You make of Node.js what you will.
Spring forces you to use Java Beans DAO objects, and to organize HTTP responses around Controller classes, to use lots of XML files to describe things that should be written in code, and on and on. The Java Bean model relies on Java annotations that perform magic functions where you write a "simple" Java class sprinkled with annotations. The system then magically instantiates these Beans somehow and on and on. It's really nauseating thinking about how many layers of abstraction are going on, and the computational/complexity overhead entailed by something as innocuous-seeming as the @Autowired annotation. How does Spring magically figure out how to find the value it is autowiring into place? And why is it so hard to just explicitly write the code in the first place?
There are MVC frameworks written on top of Node.js. The better question would have been - how does Express (or another framework) compare against Spring? But even that's not good since Express itself is not MVC-oriented.
In any case, the frameworks available for Node.js tend to be streamlined, easy to program, straightforward, etc. The JavaScript language helps a lot with this since it is so much easier to program in JavaScript than in Java.
Way-back-when, what was my argument that Java is superior? The Java language actively helps you catch certain kinds of errors because of strict type-checking of all kinds of things. Fields in objects, method parameters, and basically everything else you touch has to be rigidly and correctly typed. In JavaScript you're using anonymous objects and anonymous functions all over the place, with no data typing, and it would seem like a nightmare of potential problems.
But the impact of strict typing is an onerous burden of coming up with data types for everything you touch. What if you have two methods communicating data -- data that will ONLY be exchanged PRECISELY between these two methods? In Java you still have to define a Class to store that data, and depending on the shape of that data the Class might be complex. In JavaScript you simply throw the data into an anonymous object, and make sure the two functions are written correctly to deal with the object. An example is the JSON Mapper I mentioned earlier -- 600 lines of code to manage an object with 20 fields. Bah.
In Express/Node.js it's this simple
res.send({
field1: value1, field2: value2, field3: value3, ...
});
The result is the same - JSON sent to the caller. Which is easier to write, easier to maintain, easier to understand?